There are basically two ways to validate a situation in Java and complain when something unexpected happens. It’s either an exception or an assertion. Technically, they are almost the same, but there are some small differences. I believe that exceptions are the right way to go in all situations and assertions should never be used. Here’s why.
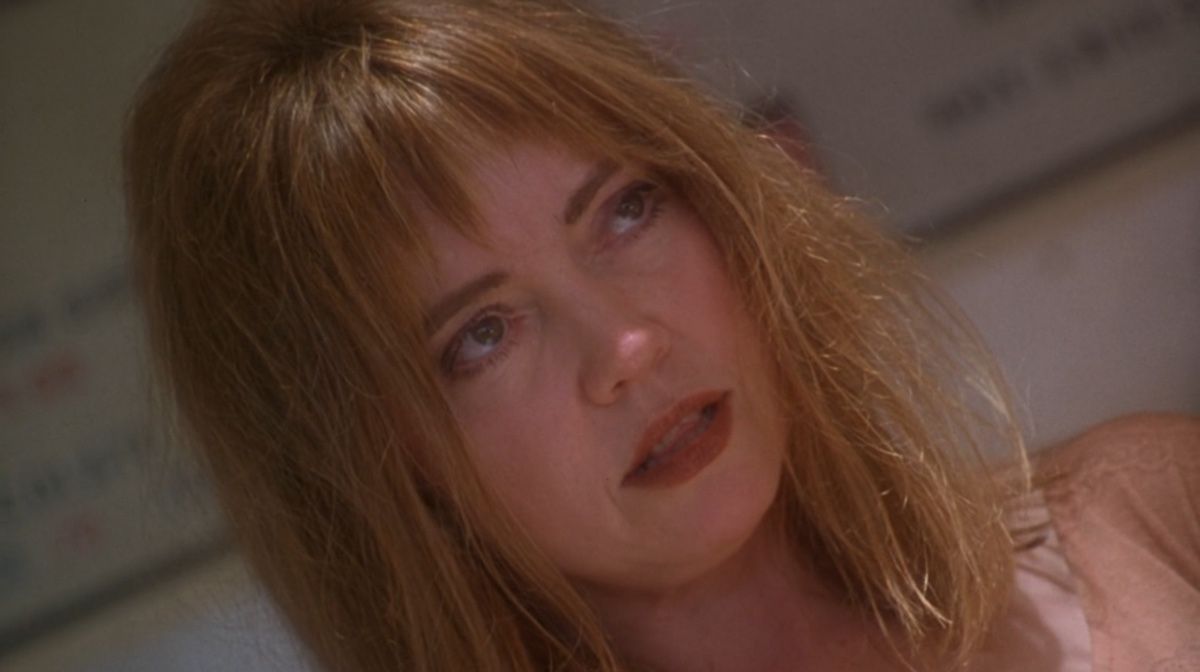
Let’s see what happens when an assertion is triggered. Say that this is our code:
public class Main {
public static void main(String... args) {
assert true == false : "There is a problem";
System.out.println("Hello, world!");
}
}
Save this code to Main.java
and compile:
$ javac Main.java
Then run it:
$ java Main
Hello, world!
The assertion wasn’t triggered. It was ignored. Now run it with an -enableassertions
flag:
$ java -enableassertions Main
Exception in thread "main" java.lang.AssertionError: There is a problem
at Main.main(Main.java:3)
This is the first difference between exceptions and assertions. Exceptions will always be thrown, while assertions are not enabled by default. They are supposed to be turned on during testing and turned off in production. The assertion caused the runtime exception AssertionError
. But hold on; it’s not a RuntimeException
. It extends Error
class, which extends Throwable
. This is the second difference. I don’t know of any other differences.
I would recommend not to use assertions … ever. Simply because I strongly believe in the Fail Fast approach. I think bugs must be visible not only during testing but also in production. Moreover, I believe making bugs visible in production is very important if you want to achieve a high-quality product.
Thus, no assertions. They are simply a flawed and outdated feature in Java (and some other languages).
Do you use assertions? #java
— Yegor Bugayenko (@yegor256) March 22, 2020